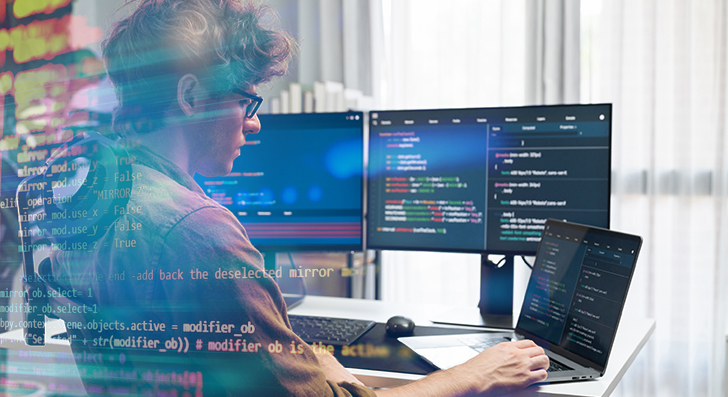
Scalability indicates your application can deal with growth—extra people, a lot more information, and much more traffic—without breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and useful guideline that will help you get started by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability just isn't anything you bolt on later on—it ought to be element within your approach from the beginning. Lots of programs are unsuccessful after they mature quickly because the initial structure can’t cope with the extra load. Being a developer, you need to Consider early regarding how your technique will behave stressed.
Commence by coming up with your architecture to get flexible. Stay away from monolithic codebases where by every little thing is tightly linked. Instead, use modular design and style or microservices. These styles break your app into smaller, independent areas. Each module or services can scale By itself without influencing The complete technique.
Also, contemplate your databases from day one particular. Will it need to manage a million buyers or simply just 100? Select the right style—relational or NoSQL—determined by how your information will grow. Approach for sharding, indexing, and backups early, Even though you don’t require them still.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective under present situations. Think of what would come about If the person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use style designs that help scaling, like concept queues or occasion-driven methods. These assist your app take care of more requests without having receiving overloaded.
When you Create with scalability in mind, you're not just getting ready for achievement—you might be lessening long term complications. A well-planned process is simpler to keep up, adapt, and expand. It’s far better to arrange early than to rebuild afterwards.
Use the Right Database
Deciding on the correct database is really a important part of setting up scalable purposes. Not all databases are designed the exact same, and using the Incorrect you can sluggish you down or even induce failures as your application grows.
Begin by understanding your information. Can it be hugely structured, like rows within a table? If Certainly, a relational databases like PostgreSQL or MySQL is an effective suit. These are typically sturdy with relationships, transactions, and consistency. They also support scaling techniques like read replicas, indexing, and partitioning to handle additional targeted traffic and info.
In case your details is more adaptable—like consumer activity logs, product or service catalogs, or paperwork—consider a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing huge volumes of unstructured or semi-structured details and will scale horizontally much more quickly.
Also, think about your examine and write designs. Are you presently carrying out numerous reads with fewer writes? Use caching and read replicas. Are you dealing with a significant write load? Explore databases which will handle large produce throughput, or even occasion-dependent details storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel in advance. You might not need Superior scaling characteristics now, but deciding on a databases that supports them implies you gained’t will need to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your obtain styles. And always watch databases performance as you expand.
In a nutshell, the best databases will depend on your application’s framework, pace desires, and how you anticipate it to develop. Consider time to pick sensibly—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, every single modest delay adds up. Poorly created code or unoptimized queries can slow down performance and overload your system. That’s why it’s important to Establish successful logic from the beginning.
Start out by producing clear, straightforward code. Steer clear of repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy a single performs. Keep your capabilities limited, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also long to operate or employs an excessive amount of memory.
Future, have a look at your database queries. These typically slow points down over the code alone. Ensure Each individual query only asks for the data you truly require. Stay clear of Pick *, which fetches everything, and as a substitute select distinct fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, In particular across significant tables.
Should you see exactly the same facts being requested time and again, use caching. Store the outcome quickly using resources like Redis or Memcached therefore you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with significant datasets. Code and queries that function fantastic with one hundred data could possibly crash when they have to handle 1 million.
In a nutshell, scalable apps are rapidly applications. Keep the code limited, your queries lean, and use caching when required. These measures support your software keep clean and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra users and more visitors. If almost everything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout many servers. In place of one particular server undertaking each of the perform, the load balancer routes customers to different servers dependant on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Instruments like Nginx, HAProxy, or cloud-primarily based options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it might be reused speedily. When consumers ask for the exact same details again—like an item webpage or even a profile—you don’t have to fetch it within the database every time. You can provide it in the cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
2. Client-aspect caching (like browser caching or CDN caching) stores static information near the consumer.
Caching cuts down database load, increases speed, and can make your app far more efficient.
Use caching for things that don’t improve usually. And usually ensure that your cache is updated when knowledge does improve.
In a nutshell, load balancing and caching are very simple but potent instruments. Together, they help your application handle far more buyers, stay rapidly, and Get better from troubles. If you propose to grow, you may need both of those.
Use Cloud and Container Tools
To create scalable apps, you would like resources that allow your app improve easily. That’s exactly where cloud platforms and containers are available in. They provide you adaptability, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term capability. When site visitors will increase, it is possible to insert much more sources with only a few clicks or instantly making use of automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply companies like managed databases, storage, load balancing, and protection instruments. It is possible to target constructing your app rather than handling infrastructure.
Containers are another crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be simple to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most popular Device for this.
When your application employs numerous containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual elements of your application into providers. You can update or scale sections independently, and that is great for general performance and dependability.
To put it briefly, working with cloud and container resources usually means it is possible to scale fast, deploy quickly, and recover promptly when issues transpire. If you'd like your application to develop devoid of limits, start off using these instruments early. They conserve time, lessen risk, and enable you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location troubles early, and make improved decisions as your app grows. It’s a essential Component of building scalable methods.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and companies are accomplishing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application much too. Keep watch over how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a support goes down, you ought to get notified right away. This can help you correct troubles rapidly, typically ahead of end users even observe.
Monitoring is additionally helpful whenever you make modifications. In the event you deploy a brand new feature and find out a spike in glitches or slowdowns, it is possible to roll it back again prior to it causes real destruction.
As your application grows, site visitors and information raise. With no monitoring, you’ll pass up signs of trouble right until it’s way too late. But with the proper check here applications in position, you stay on top of things.
In brief, checking aids you keep your application reliable and scalable. It’s not almost spotting failures—it’s about being familiar with your program and making sure it really works well, even under pressure.
Final Feelings
Scalability isn’t just for significant firms. Even small apps have to have a powerful Basis. By creating diligently, optimizing properly, and utilizing the right instruments, you are able to Make apps that increase effortlessly without having breaking stressed. Begin smaller, think massive, and Establish wise.